Inner Loop vs Outer Loop: Understanding Their Differences and Applications
In the realm of software engineering, understanding the intricacies of loops is crucial for efficiency and optimization. Loops are fundamental programming constructs that enable us to execute a block of code repeatedly. Among the various types of loops, inner loops and outer loops play significant roles in how we organize and execute our programs. This article will delve into the definitions, differences, applications, and implications of inner and outer loops, providing insights for better coding practices.
Defining the Concepts: Inner Loop and Outer Loop
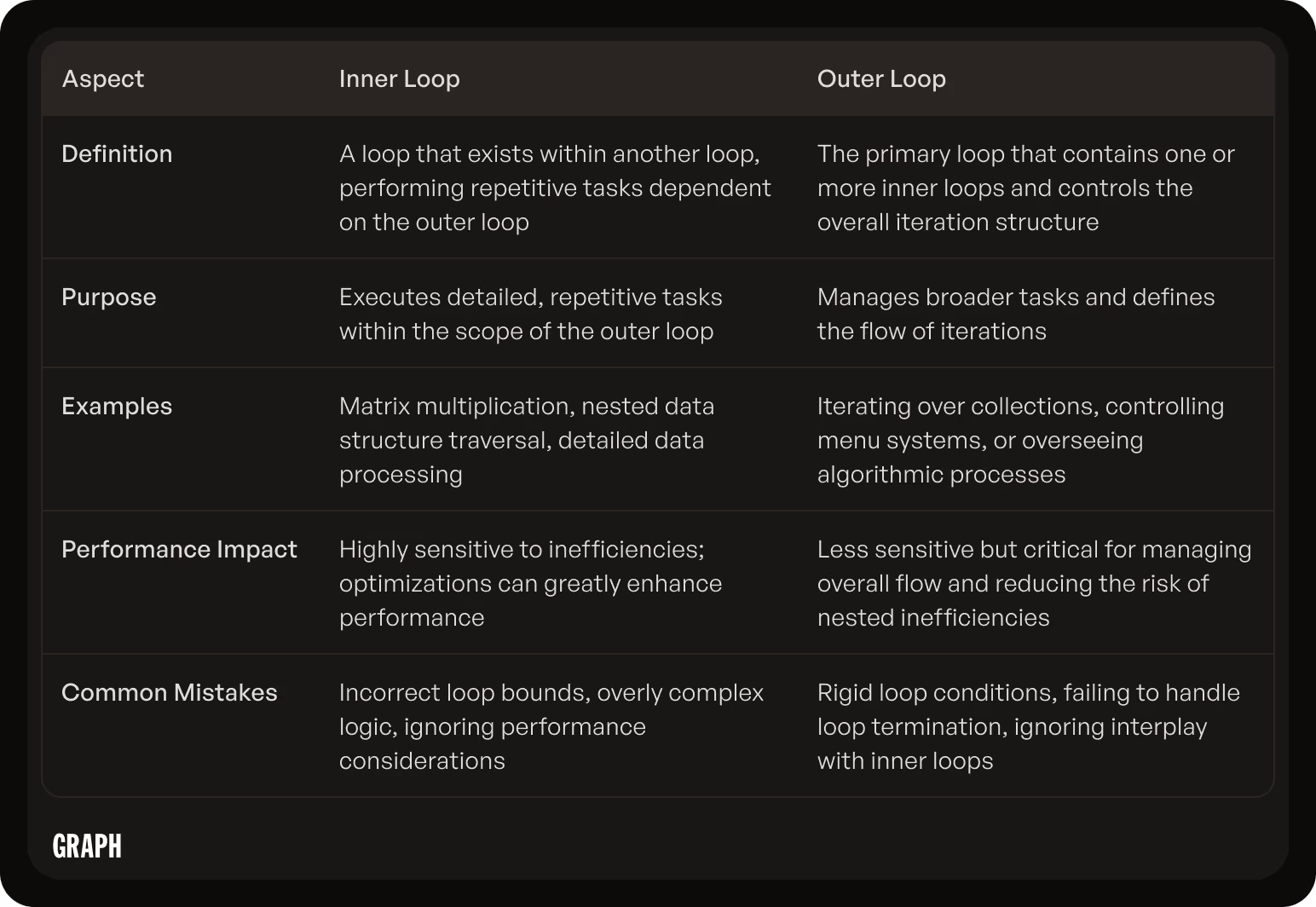
What is an Inner Loop?
An inner loop is defined as a loop that exists within another loop, often referred to as the outer loop. The purpose of the inner loop is typically to perform repetitive tasks that are dependent on the iteration of the outer loop. For instance, in a nested structure, the inner loop will execute its code block entirely for each execution of the outer loop. This configuration allows for complex operations to be executed efficiently.
One common example of an inner loop can be seen in algorithms that require multi-dimensional data processing, such as matrix multiplication. The inner loop handles the multiplication and addition operations for each element, while the outer loop iterates through rows and columns, maintaining organization in the data structure. Moreover, inner loops can also be utilized in scenarios such as searching through nested data structures, where each level of nesting requires its own set of operations. This makes inner loops incredibly versatile, as they can adapt to a variety of programming challenges, from simple iterations to more complex algorithmic tasks.
What is an Outer Loop?
The outer loop is the primary loop that encases one or more inner loops. It typically dictates the overall structure and flow of the iteration process. The outer loop is responsible for initiating the sequence of operations, while inner loops handle the specific tasks required within each iteration of the outer loop.
For example, when processing a list of items, the outer loop may iterate through the collection of items, while an inner loop can handle operations like filtering or transforming each item based on certain criteria. This hierarchical structure allows for organized and manageable code. Additionally, outer loops can be instrumental in controlling the overall execution of algorithms that require multiple passes over data, such as sorting algorithms. In these cases, the outer loop ensures that the entire dataset is processed multiple times until the desired order is achieved, showcasing the critical role that outer loops play in algorithm efficiency and effectiveness.
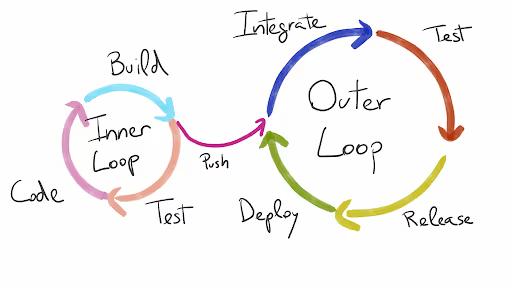
The Fundamental Differences Between Inner and Outer Loops
Structural Differences
The structural differences between inner and outer loops can be articulated by understanding their hierarchical relationship. The outer loop encompasses the overall process, while the inner loop functions as a subsidiary task within that process.
- The outer loop controls the primary flow of execution.
- The inner loop is executed multiple times for every single iteration of the outer loop.
- When analyzing code, outer loops generally appear first in terms of placement within the logic framework.
In many programming languages, the syntax and structure of loops can vary significantly, but the fundamental concept of nesting remains consistent. For instance, in languages like Python, the indentation level indicates the hierarchy of loops, making it visually clear which loop is an outer loop and which is an inner loop. This visual distinction can aid developers in quickly understanding the flow of control within their code, thereby reducing the likelihood of errors that arise from mismanaged loop structures.
Functional Differences
Functionally, the inner and outer loops serve distinct roles. The outer loop captures broader tasks that require multiple repetitions, whereas the inner loop's focus is more granular. This is especially pertinent in performance-sensitive applications.
- The outer loop may determine the scope of processing, such as iterating through each record in a dataset.
- Within that iteration, the inner loop can execute detailed operations, like validating or modifying data points.
This division of labor allows software engineers to manage complexity and enhance the readability of their code. Understanding these functional differences can lead to better optimization practices. For example, in scenarios where the inner loop performs a heavy computation, developers might consider optimizing that section of code to minimize the overall execution time. Techniques such as loop unrolling or caching results from previous iterations can significantly enhance performance, especially when the outer loop is expected to run a large number of times.
Moreover, the interplay between inner and outer loops can also affect resource management within an application. If the inner loop is responsible for resource-intensive tasks, such as database queries or network calls, it is crucial to ensure that these operations are efficient to prevent bottlenecks. This might involve implementing asynchronous processing or leveraging batch operations to reduce the overhead incurred during each iteration of the outer loop. By carefully considering the functional roles of each loop, developers can create more efficient and maintainable code that scales effectively with increasing data sizes or user demands.
Exploring the Applications of Inner and Outer Loops
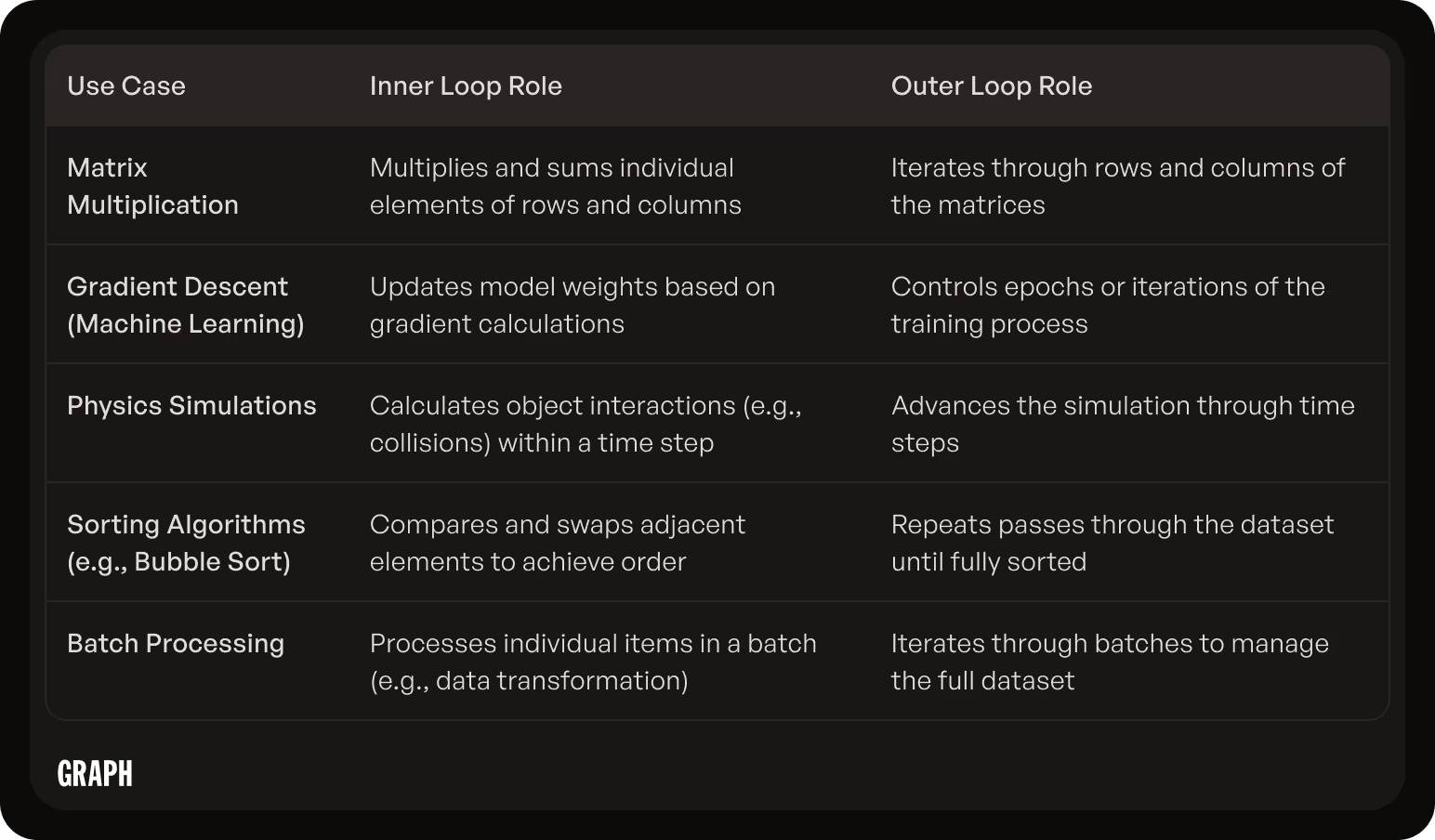
Common Uses of Inner Loops
Inner loops are often utilized in scenarios requiring repetitive and localized computations. Common applications include:
- Data processing tasks, where each element must undergo a similar transformation.
- Nested data structures, such as lists of lists, where operations must be applied iteratively across dimensions.
- Algorithms like sorting and searching, where repeated checks or operations must occur within an outer context.
In addition to these applications, inner loops play a crucial role in machine learning algorithms, particularly during the training phase. For instance, in gradient descent, inner loops are used to update model weights iteratively based on the computed gradients. This repetitive adjustment allows the model to converge towards optimal performance. Moreover, inner loops can also be found in graphics programming, where pixel manipulation often requires nested iterations to achieve effects like blurring or sharpening images, demonstrating their versatility across various domains.
Common Uses of Outer Loops
Outer loops are integral to a variety of programming tasks as well. They typically handle overarching processes needed for:
- Iterating over collections, such as arrays or lists, to apply processes across all elements.
- Controlling user-driven processes, such as maintaining a menu system until a termination condition is met.
- Managing state in multi-threaded applications, where operations must reoccur based on changing conditions.
Furthermore, outer loops are essential in simulations and modeling, where they can control the progression of time or iterations through a series of events. For example, in a physics simulation, an outer loop might represent each time step, allowing for the calculation of motion and interactions among objects in a virtual environment. This structure not only enhances readability but also allows for easier debugging and modification of the simulation parameters. Additionally, outer loops can be instrumental in batch processing tasks, where entire datasets are processed in chunks, optimizing performance and resource management in data-intensive applications.
By leveraging both inner and outer loops appropriately, developers can construct highly efficient algorithms tailored to specific tasks.
The Interplay Between Inner and Outer Loops
How Inner and Outer Loops Work Together
Understanding how inner and outer loops interact can revolutionize your programming practice. When both types of loops function in harmony, they allow for complex tasks to be executed efficiently. For example, when handling large datasets, using an outer loop to iterate through records while employing inner loops for detailed processing can significantly reduce the complexity of the code.
Additionally, this interaction plays a crucial role in algorithm optimization, especially in nested iterations. Coders must be mindful of how many times each loop gets executed within one another to avoid performance pitfalls. This awareness not only aids in writing cleaner code but also enhances the overall performance of applications, particularly in data-heavy environments where speed is paramount. Consider scenarios such as data analysis or machine learning, where the efficiency of loop structures can directly influence the speed of model training and data processing.
The Impact of Loop Nesting
Nesting loops, while useful, can also lead to performance challenges. The more you nest inner loops, the greater the potential impact on runtime. Since inner loops execute multiple times for each iteration of the outer loop, excessive nesting can lead to exponential growth in execution time. This exponential growth can become a critical bottleneck, especially in applications that require real-time processing or quick response times.
Reviewing loop nesting is vital for performance tuning. Inefficiencies can often be mitigated through techniques like loop unrolling or by restructuring code to minimize the effective depth of nesting. Moreover, employing alternative data structures, such as hash tables or sets, can reduce the need for deeply nested loops by allowing for faster lookups and operations. By strategically analyzing the logic behind nested loops and considering the overall algorithmic complexity, developers can achieve significant improvements in both performance and maintainability of their codebases.
Choosing Between Inner and Outer Loops
Factors to Consider
Choosing between using an inner loop or an outer loop depends on several factors, including:
- The nature of the data being processed: Multi-dimensional data often necessitates nested loops.
- The complexity of operations required within each iteration: Consider if repetitive tasks can be optimized.
- Performance considerations: Evaluate whether the additional overhead of nested loops is justified.
Moreover, the size of the dataset can significantly influence your choice. For smaller datasets, the performance hit from nested loops may be negligible, allowing for clearer code structure. However, as data size increases, the inefficiencies of nested loops can become pronounced, leading to longer execution times. It's also important to consider the data access patterns; if data is stored in a way that favors sequential access, a single loop might be more efficient than a nested approach.
Making the Right Choice for Your Project
Ultimately, making the right choice involves a balance between clarity and performance. It's vital to write code that is not only functional but also maintainable. When deciding on loop structure, consider:
- The likelihood of code modifications in the future.
- The overall performance impact in the context of your application's requirements.
Tools like code profilers can help visualize and quantify the execution time of various loops, guiding your decision-making process. Additionally, it may be beneficial to conduct benchmark tests with different loop configurations to see how they perform under realistic conditions. This empirical approach can provide insights that theoretical considerations alone may not reveal. Furthermore, consider the readability of your code; sometimes, a slightly less efficient loop may be preferable if it makes the code significantly easier to understand for future developers or even for yourself down the line.
Potential Pitfalls and How to Avoid Them
Common Mistakes with Inner Loops
Inner loops, while powerful, can introduce pitfalls if not structured thoughtfully. Common mistakes include:
- Unintentional infinite loops due to incorrect loop bounds.
- Overly complex inner logic that leads to decreased readability.
- Ignoring performance considerations, resulting in slower runtimes.
To mitigate these issues, it's crucial to establish clear and concise loop conditions. For instance, using descriptive variable names and comments can greatly enhance the readability of the loop's logic, making it easier for others (or yourself in the future) to understand the intended function. Additionally, employing debugging techniques, such as logging the loop's progress or using breakpoints, can help identify potential infinite loops before they become problematic. Furthermore, considering the algorithmic complexity of the inner loop can lead to significant performance improvements, especially in scenarios involving large datasets.
Common Mistakes with Outer Loops
Outer loops also come with their own set of challenges. Mistakes to watch for include:
- Coding outer loops that are too rigid and do not accommodate all scenarios.
- Failing to break out of a loop when needed, which can lead to resource consumption issues.
- Not considering the interaction between outer and inner loops, leading to unexpected behavior.
One effective strategy to avoid these pitfalls is to implement flexible loop conditions that can adapt to various inputs or states. This adaptability not only enhances the robustness of your code but also minimizes the risk of encountering edge cases that could disrupt execution. Additionally, it's beneficial to regularly review and refactor your loops to ensure they remain efficient and maintainable. By keeping an eye on the interplay between outer and inner loops, you can better manage the flow of data and logic, ultimately leading to more reliable and efficient code execution.
Conclusion: Harnessing the Power of Inner and Outer Loops
In conclusion, understanding the distinctions and applications of inner and outer loops is of paramount importance for efficient software engineering. By leveraging the structural and functional strengths of each type of loop, developers can create optimized, maintainable, and efficient code.
From managing complex data sets to optimizing performance, the right use of loops can make a significant difference in the design of algorithms and the overall execution of programs. By avoiding common pitfalls and being mindful of loop nesting and interaction, engineers can harness the full power of inner and outer loops in their programming endeavors.